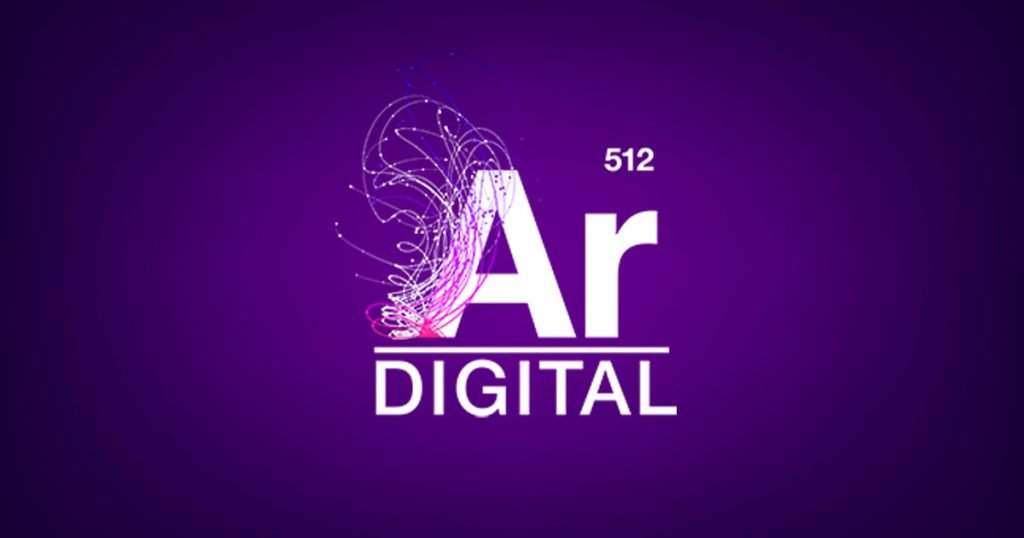
‘Twas the night before launch day
‘Twas the night before launch and all through that dayNot a creature had stirred not even QA The requirements had been collected from the users with careIn the hopes that
Skip to content
‘Twas the night before launch and all through that dayNot a creature had stirred not even QA The requirements had been collected from the users with careIn the hopes that
The decision to use a requirements tool is dependent on a number of factors: size of project, number of system interlocks, global teams, specific business objectives tied to real dollar
The decision to use a requirements tool is dependent on a number of factors: size of project, number of system interlocks, global teams, specific business objectives tied to real dollar
Companies that make the switch from Waterfall methodologies to Agile struggle with requirements documentation. How much documentation do we need? What kind? When should this documentation be created? Who is
Recently, I attended a luncheon with a diverse group of people in the IT industry–coders, program managers, product managers, business analysts, and architects. The topic of the luncheon is not
Does anyone know the history of the shall statement in requirements? I hear people now and then complain about that word. I personaly don’t like it as it’s not a
There’s a chance that during this time of year, when gathering with friends and family you haven’t seen in ages, or with people who just simply forget everything about you
ArgonDigital is excited to announce that our resident authors, Joy Beatty and Tony Chen, of Visual Models for Software Requirements have been recognized for their book they released last year. The
I will be presenting “What is a Requirement” at the Texas Gulf Coast Chapter (TGCC) of INCOSE on July 17 at Jacobs Technology (2224 Bay Area Blvd. – at Space
ArgonDigital offered our 1- day “Writing Good Requirements Seminar“ in Denver, Colorado on April 9, 2013. With the right approach, knowledge, tools and techniques, you can deliver projects on-time and
The year is 2014 C.E. Two rival Tribes are converging on a grassy field littered with tables, in modern-day Stockholm. There is tension in the cold, winter air. The Squads
We’ve all been there. You are trying to stream the new season of Arrested Development, but your brand new router refuses to connect. You’ve unplugged and then re-plugged the device
Don’t miss out on this opportunity
and request a conversation today!
Cookie | Duration | Description |
---|---|---|
cookielawinfo-checkbox-analytics | 11 months | This cookie is set by GDPR Cookie Consent plugin. The cookie is used to store the user consent for the cookies in the category "Analytics". |
cookielawinfo-checkbox-functional | 11 months | The cookie is set by GDPR cookie consent to record the user consent for the cookies in the category "Functional". |
cookielawinfo-checkbox-necessary | 11 months | This cookie is set by GDPR Cookie Consent plugin. The cookies is used to store the user consent for the cookies in the category "Necessary". |
cookielawinfo-checkbox-others | 11 months | This cookie is set by GDPR Cookie Consent plugin. The cookie is used to store the user consent for the cookies in the category "Other. |
cookielawinfo-checkbox-performance | 11 months | This cookie is set by GDPR Cookie Consent plugin. The cookie is used to store the user consent for the cookies in the category "Performance". |
viewed_cookie_policy | 11 months | The cookie is set by the GDPR Cookie Consent plugin and is used to store whether or not user has consented to the use of cookies. It does not store any personal data. |