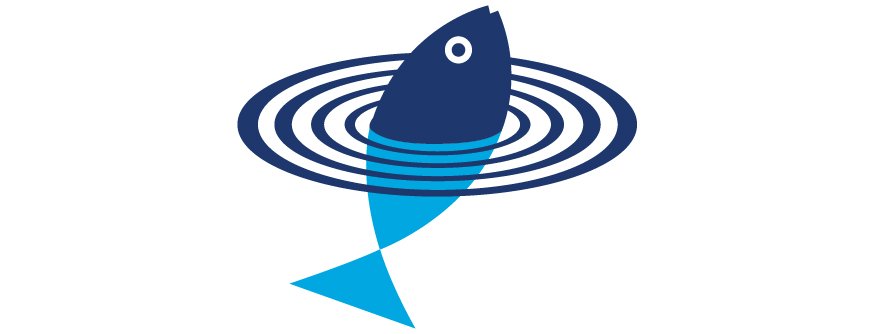
@font-face: The Easiest Way to Use Custom Fonts on Your Web Site
Introduction Creative typography has long been unavailable to most designers on the web. We’re stuck with the same old boring fonts installed on all machines: Arial, Times New Roman, Georgia,